Getting tickets from Zendesk using JavaScript
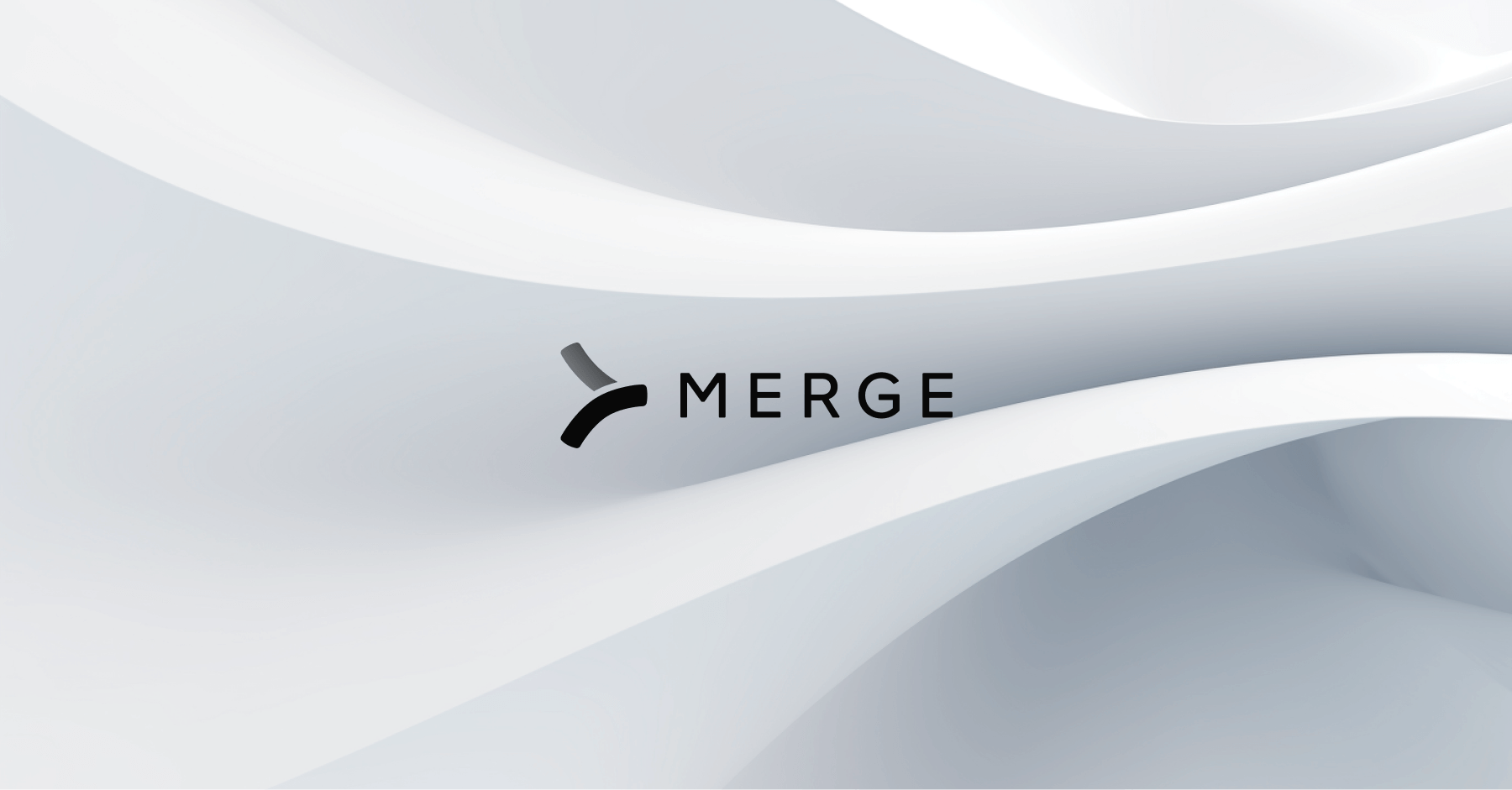
This article is part of a series on building product integrations with third-party platforms.
You can think of a ticketing system like Zendesk as the control center for customer support. It's a one-stop-shop where businesses monitor and work on customer issues, filed as "tickets". Zendesk, along with similar platforms, offers tools like automation, analytics, and integration capabilities to make the customer service process smoother and boost customer satisfaction.
We frequently see use cases where developers need to access tickets from a client’s Zendesk system (think of assessing customer service performance, finding recurring problems, or even automating specific responses). The Zendesk API is useful here by allowing engineers to integrate tickets directly into their own apps or databases.
In this tutorial, we’ll dig into the Zendesk API and cover:
- Setting up the authentication process
- How to extract normalized data from Zendesk including get requests to pull tickets
- An alternative to building integrations one at a time by using a single API endpoint
{{blog-cta-100+}}
Setting up the authentication process
In order to access the Zendesk API, the requests you send need to be authenticated. This involves including a specific header in your request, which is formatted as `Authorization: Basic {Email Address}/token:{API-KEY}`. This string is a base64 encoded version of your email address, followed by '/token', and then your API key. This header tells Zendesk that your request is authorized, allowing you to successfully pull tickets from their API.
Replace `YOUR_EMAIL`, `YOUR_API_TOKEN`, and `YOUR_ZENDESK_DOMAIN` with your actual email, API token, and Zendesk domain respectively. This code uses Node.js built-in modules - `https` for making an HTTP request, and `buffer` for encoding your email and token into base64 format.
How to get tickets from Zendesk
The code above prepares an options object that includes the request details - the hostname, path, HTTP method, and headers. The authorization header is included as specified, where the email and token are encoded in base64.
Then, it sends a GET request to the Zendesk API. When the response data is received, it aggregates the data chunks into a complete JSON string. Once all data has been received, it parses the JSON string into a JavaScript object and logs the tickets to the console. If the `has_more` field in the `meta` object of the response is true, it sets the path in the options object to the `next` link and sends another GET request. If there's an error with the request, it logs the error message to the console. Finally, it calls `req.end()` to signify the end of the request.
Here's an example of an individual item returned by this API Endpoint:
Related: The steps for reading API documentation
Use a unified API to offer a variety of ticketing integrations
In this article, we walked through the process of leveraging Javascript to connect with Zendesk and retrieve tickets. But what if your clients use a variety of ticketing systems?
You can integrate your product with all of your clients' ticketing solutions to sync tickets—among other types of data—by leveraging Merge, the leading Unified API solution.
Simply build to Merge's ticketing Unified API to offer 30+ ticketing integrations. You'll also be able to access Merge's robust Integrations Management features to identify and troubleshoot issues quickly and successfully.
You can learn more about Merge by scheduling a demo with one of our integration experts.