Understanding the difference between RPC and REST for web APIs

Software developers love to argue about the best technologies, techniques, development lifecycle methods, and everything in between. One of the big battles developers are currently having is between RPC and REST APIs -- the two most common architectural styles used to design and develop web APIs.
RPC has been around for decades, and at face value, it is straightforward due to the lack of rigid conventions RPC requires. RPC stands for “remote procedure call.” One server can execute a function that looks and seems to act similar to a local function. Yet behind the scenes, it is communicating with another server over the network. Slack’s API is perhaps the most well-known example of an RPC-style web API.
REST, on the other hand, stands for “representational state transfer.” In REST APIs, your system will represent its current state via an API through “resources.” Resources are abstractions of some collection of objects. For example, your API might present a <code class="blog_inline-code">/schedules</code> resource for scheduling software. In an e-commerce web API, you may have resources like <code class="blog_inline-code">/products</code>, <code class="blog_inline-code">/orders</code>, etc.
It’s important to understand the difference between the two styles of designing and building web APIs as they affect, in different ways, your API’s performance, client discovery, maintainability, versioning, and more. Some APIs are more action oriented and need those actions to be performed very quickly (like processing real-time data). Other APIs need a semantics-oriented style that allows clients to use standard conventions to easily browse through the API functions.
In this article, you’ll learn more about these two API styles and how they each work. More importantly, you’ll learn about why and when using one style over the other makes sense and how they can benefit your APIs. There may even be times that having RPC and REST web APIs in your business makes sense, depending on the focus and needs of the specific APIs and their business functions.
RPC APIs
RPC APIs are the more natural style of API. You create a function that does some calculation, or it may simply return some data. RPC APIs usually stick to using the GET and POST HTTP verbs.
Imagine an API endpoint that will return data for all the orders that you may want to view:
An endpoint to create a new order might look like this:
Again, this style is straightforward, and it is what you might consider the more natural approach to designing an API.

Advantages of RPC
Here are some advantages of RPC APIs:
- They’re simple to implement
- They’re easy to extend—you just add another function/endpoint
- They’re performance oriented—RPC is a great option when performance and/or low-latency writes are critical
Because of these benefits, RPC is the ideal choice when you need focused, action-oriented API endpoints that can be individually tweaked and optimized. We recently discussed with Ashby their reasoning for selecting RPC-style for their public API, and a lot of these advantages were referenced in their decision-making.
Disadvantages of RPC
Here are some disadvantages of RPC:
- RPC involves more coupling—its methods can be a leaky abstraction
- It is difficult to discover methods and predict what the API’s semantic conventions are
- Code is harder to keep “clean” since every action is a separate method call. This can lead to an explosion of separate endpoints that are all very similar
- Developers are often-times less familiar with RPC, and so RPC-style APIs oftentimes requires more developer support
Because of RPC’s disadvantages, you may not want to use RPC if you are building an API where one critical focus is that it can be easily browsed and discovered by your consumers.

When to Use RPC
Here are a few real-world use cases for when using RPC might make sense for your APIs:
- RPC is a great option for synchronous service-to-service communication. Communication between services usually needs to be fast, and RPC can give you that performance your API needs.
- When the system behind the API is task heavy or command centric (e.g., “send message,” “process that data,” “start that server,” etc.), RPC can make this straightforward.
- RPC is well suited for working with small devices that need to communicate over the network (e.g., IoT).
- Real-time data processing that requires high performance can benefit from an RPC API. Specifically, RPC can facilitate APIs that require the need for streaming, binary serialization, and multiplexing.
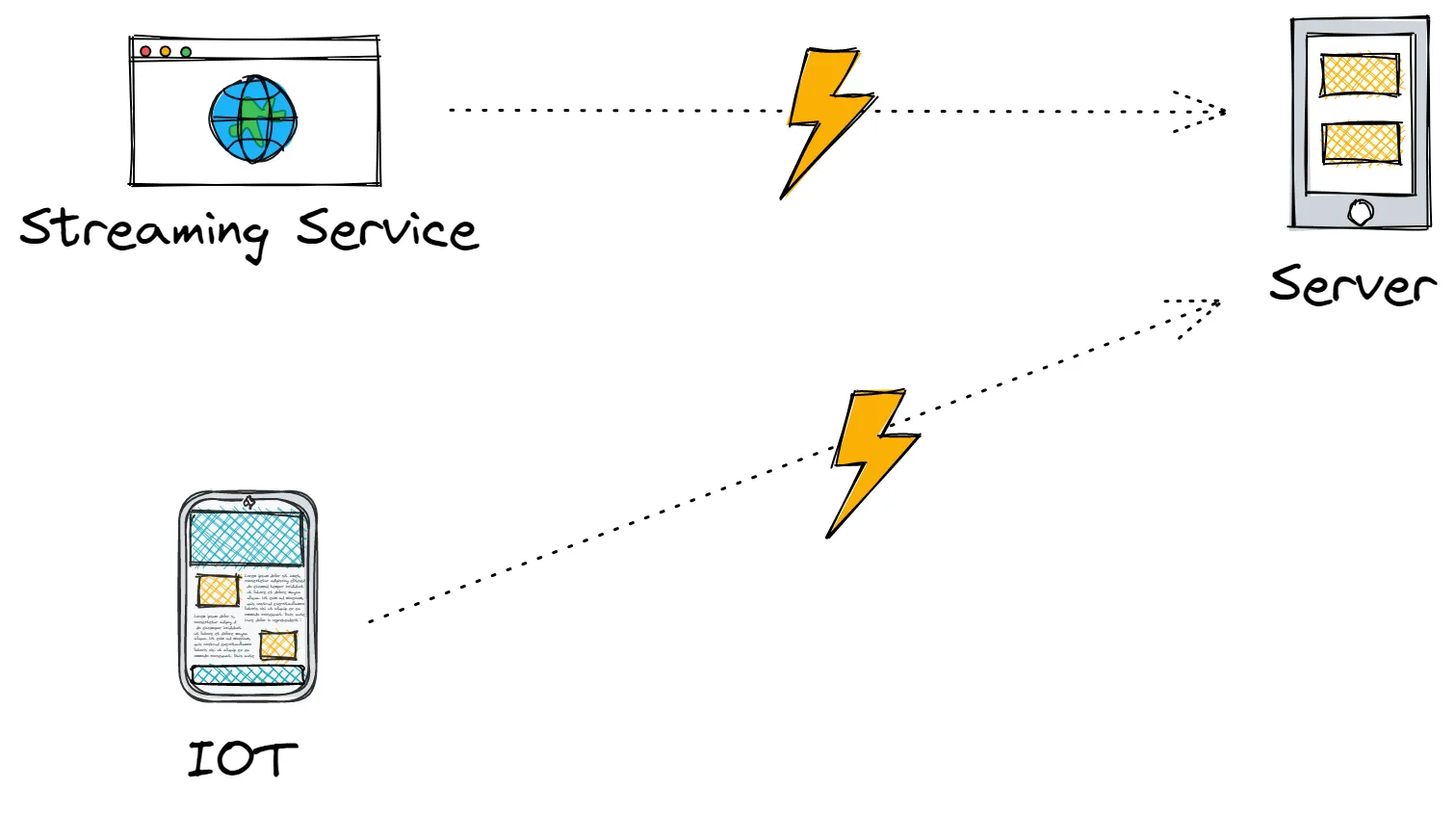
High-Performance RPC
Finally, let’s take a quick look at the biggest benefit and use case of RPC: high-performance APIs.
gRPC and Twirp are two modern protocols that are designed for building high-performance APIs. Microsoft’s comments on gRPC performance highlight the performance potential of using gRPC:
“gRPC is lightweight and highly performant. It can be up to 8x faster than JSON serialization with messages 60-80% smaller.”
While REST generally uses XML or JSON as a serialization contract, protocols like gRPC use performance-oriented serialization formats like Protocol Buffers to make sure that serialization and network payload size are well optimized.
REST APIs
The concept of REST originates from a dissertation by Roy Fielding published in 2000. REST has a few main attributes that characterize it as being stateless and having cacheable responses.
The most notable of these attributes is that REST APIs have a standard convention of how data or objects from your system will be presented to the outside world (e.g., your consumers). In REST, these objects are called resources.
Part of the standard convention is to reuse existing HTTP verbs to interact with specific resources. The following are the HTTP verbs and their functions in the context of a given resource like <code class="blog_inline-code">/orders</code>:
- GET always returns data and does not cause side effects.
- POST is used for creating resources. It can return data such as the resource it just created.
- PUT and PATCH are for updating an existing resource.
- DELETE is for deleting a given resource.
Advantages of REST APIs
Roy Fielding’s original dissertation highlights the biggest advantage of REST:
“The REST interface is designed to be efficient for large-grain hypermedia data transfer, optimizing for the common case of the Web.”
In other words, REST APIs generally accept and return generic data with decently sized responses. This allows the API to be more generic and allows the client to decide exactly what pieces of data it needs to use and how to use them.
Other advantages of REST include the following:
- REST makes it easier to discover what endpoints are available and what actions can be performed against the API.
- REST leverages HTTP more than RPC does (e.g., HTTP verbs, HTTP caching, HTTP content negotiation, etc.).
- REST is more easily extensible than RPC in that new sets of data (e.g., <code class="blog_inline-code">/book/1/author</code>) can be added as a nested REST resource. With RPC, this would become a totally separate endpoint forcing more requests to get the required data (e.g., <code class="blog_inline-code">/getBook?id=1</code> and <code class="blog_inline-code">/getAuthor?id=1</code>).
- Due to abstracting resources and the more generic response data, clients are more flexible in how they can use the API.
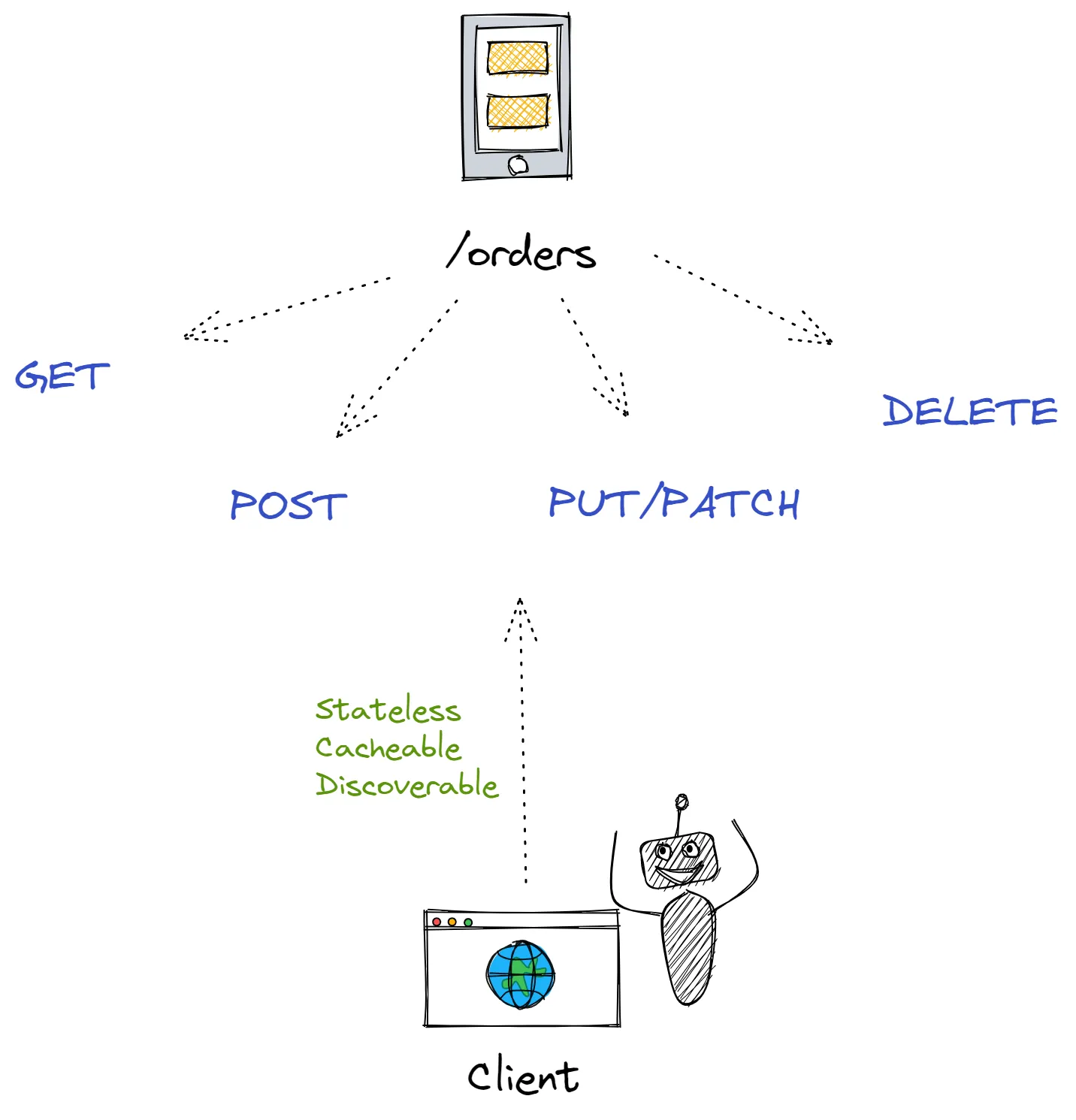
Disadvantages of REST APIs
As you might imagine, some of the disadvantages of REST are where RPC shines and vice versa. A few of REST’s limitations include the following:
- Generally speaking, REST has larger payloads. Check out this sample from GitHub’s API endpoint for Issues—you’ll notice that this one endpoint returns a lot of data for a single endpoint.
- Roy Fielding’s original dissertation highlights that a REST API is not as efficient as another style like RPC: “The trade-off, though, is that a uniform interface degrades efficiency, since information is transferred in a standardized form rather than one which is specific to an application’s needs.”
- When accessing multiple resources, you need to “jump through hoops” to get the data you need. Perhaps you first need to fetch the book resource and then make subsequent requests to additional resources to get the full data you need. Some call this “chattiness.”
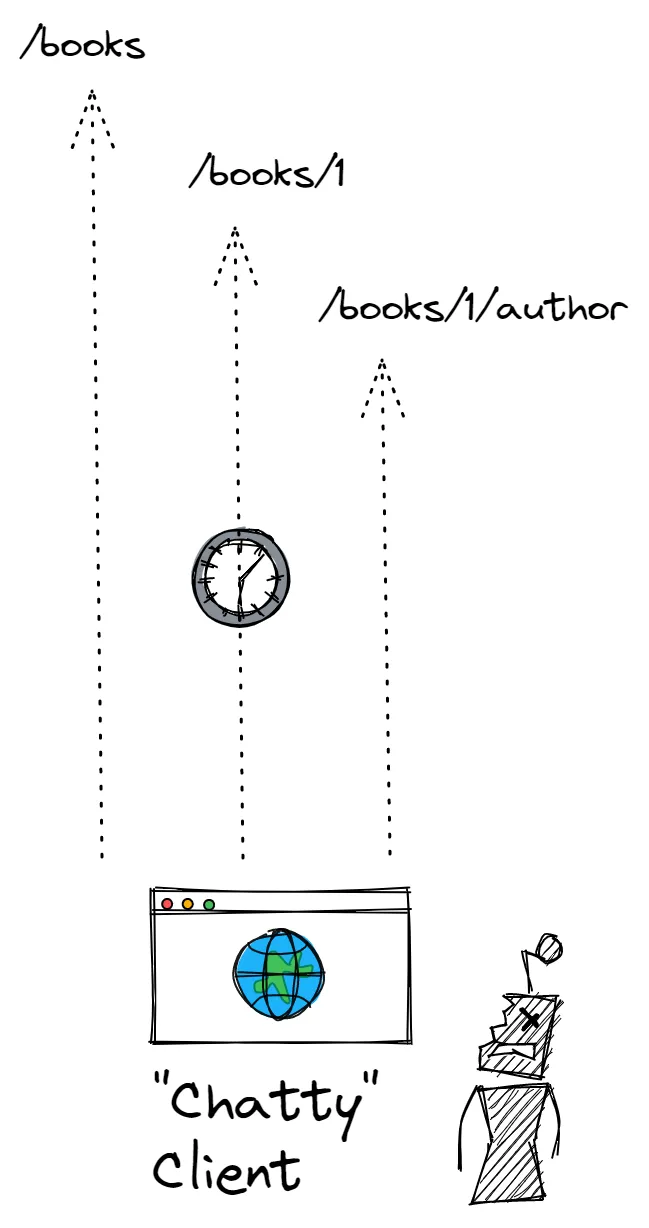
When to Use REST
REST APIs are so popular these days because of the explosion of multipurpose APIs. The same API can be used by many consumers, such as mobile applications, web applications, SDKs, internal services, and so on. Thus, here are the main use cases of the REST style of API:
- Most public APIs benefit from REST because their endpoints are easy to predict based on which resources are available. For example, a /books resource would most likely have the typical CRUD (create, update, delete) actions available for the client to interact with.
- Using REST between microservices could be appropriate whenever the service in question has many consumers and doesn’t want to be coupled to those other services.
- A public API with many consumers like mobile apps, web apps, APIs, or third-party servers would benefit from REST as it leads to a more generic interface. Consumers are forced to choose how to use the API instead of the consumers forcing you to conform to their exact needs.
- Using code-generation tools to create API documentation, SDKs, etc. is generally straightforward as typical REST conventions are followed.
- REST can be used as a platform for other API standards to be built on top of. SCIM (“System for Cross-domain Identity Management”) is an example of an API standard that uses certain REST resources (e.g., /Users and /Groups) as its foundation.

HATEOAS
When Roy Fielding created REST, hypermedia was an essential part of what REST was supposed to be. HATEOAS, which stands for “Hypermedia as the Engine of Application State,” is a piece of the original REST formulation that most APIs don’t bother to implement.
There have been debates over whether HATEOAS is necessary to have a “true” REST API. Using hypermedia in APIs is the idea that every response from your REST API will include links pointing to further actions that can be performed on the resource you are interacting with.
There are entire hierarchies that have been created to help gauge how “mature” your APIs are. For example, there’s the Richardson Maturity Model that helps gauge an API’s maturity in general—hypermedia-driven APIs being the most “mature.” Then there’s the Hypermedia Maturity Model, which gives different levels of maturity to hypermedia-driven APIs.
HATEOAS and hypermedia can enable a highly mature API that, as you’ll see, enables some helpful abilities. But it comes with a cost. It’s difficult to implement well, and it takes much more thought and time to design and build an API with this approach.
For example, a response for a request like <code class="blog_inline-code">GET /books</code> might look like the following:
Notice that each book resource has some links that allow a client to know what the next steps might be for finding out more information about each book resource. Likewise, the root object of the response has its own links attribute that tells the client how to fetch the next page of paginated results.
By including this metadata (which Roy Fielding calls “hypertext”) in all your responses, any given client can fully navigate and discover resources and actions in your API.
What if the way you paginate in your API changes? Well, you simply change the URL that the next_page link returns:
This decoupling means that you can change the implementation of your system without having to break your consumers.
Again, it’s much more work to achieve this level of abstraction. For this reason, most REST APIs do not use or implement HATEOAS—much to Roy Fielding’s dismay. But if your APIs are going to have many different kinds of clients and you want the ability to evolve your API in a decoupled fashion, then HATEOAS is an option to consider.
Conclusion
So, between RPC and REST, which is the best option for building APIs? After reading this article, hopefully, your answer is “It depends!” Each API style has its own advantages and use cases where it might be appropriate.
RPC shines in cases where you need to squeeze performance out of your API like real-time processing, streaming, and other command-oriented actions. REST, on the other hand, is the go-to for building APIs that are easily navigated—REST can decouple your consumers from your underlying system, which is especially useful when you have multiple consumers of your API, all with differing needs.
In an organization, there might be times when it’s beneficial to employ both types of API. For instance, if you have a streaming service—perhaps you stream audio or video to your customers—this might call for an RPC API. However, you might also need an API for consumers to manage their billing details, interact with their playlists, etc., which might call for a separate REST API. Netflix did something like this in 2012, and some of Twitter’s API v2 features both REST-like endpoints and RPC-style endpoints.
Merge has used the main advantage of REST APIs as the foundation of its business. Payroll and accounting APIs are notoriously confusing and difficult to use (some even still use the old SOAP protocol). Merge is a unified API for payroll, HR, recruiting, accounting, and ticketing solutions that replaces all these APIs with one common REST API.
While using RPC at times can be helpful, by using a unified REST API, Merge can abstract all these other APIs away. You only need to become familiar with one API—the Merge Unified API! Request a demo or give it a try for free!