How to GET contacts from the HubSpot CRM API in Python
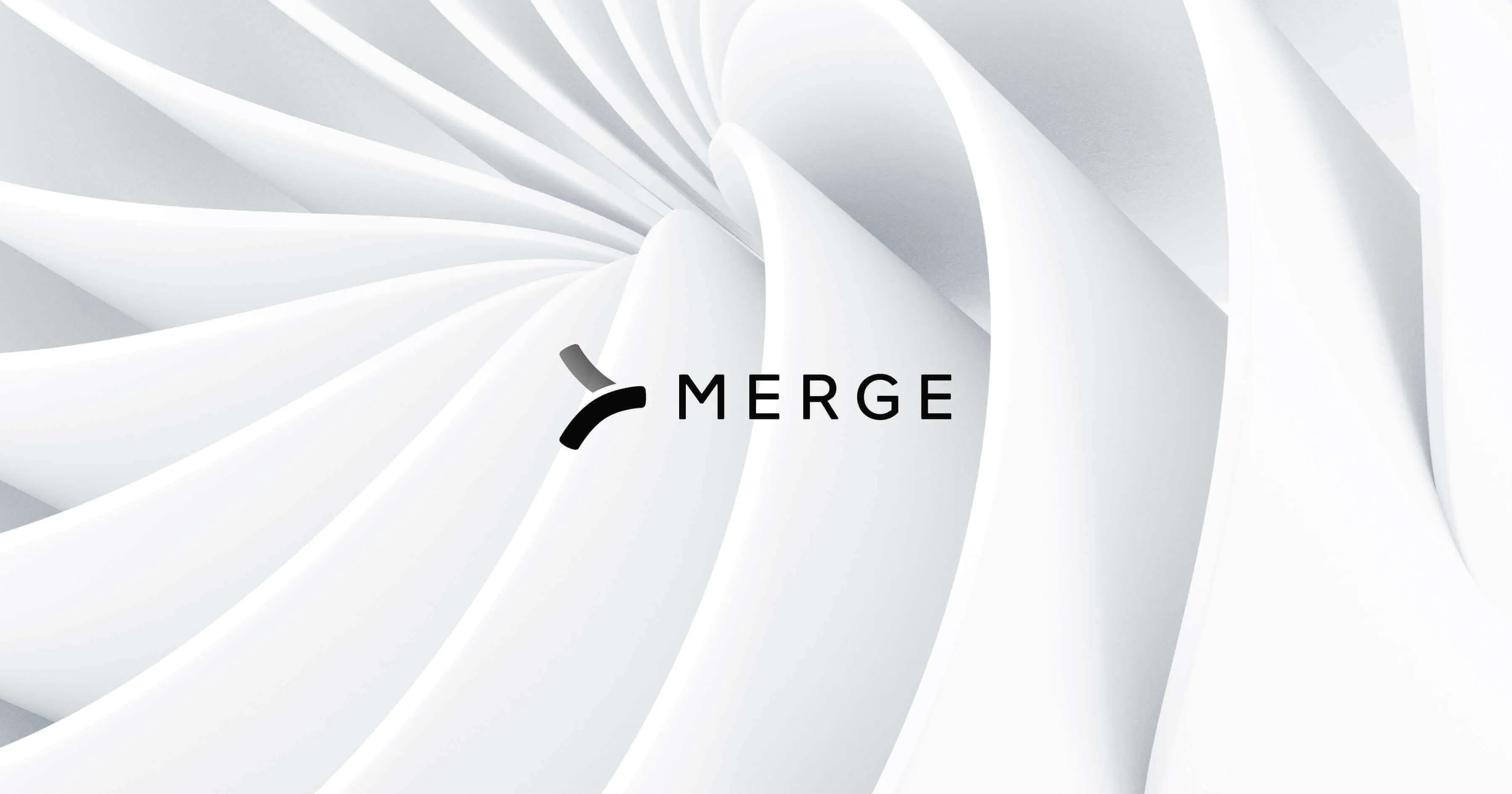
HubSpot is an all-in-one CRM platform with a comprehensive suite of tools and resources that helps businesses to connect their marketing, sales, and service efforts. Its integrated approach as well as its user-friendliness, flexibility, and top-notch customer service make HubSpot a popular choice among businesses of all sizes.
Developers may need to integrate with HubSpot to access and manage customer data, such as contacts, to personalize and automate marketing efforts. For example, a developer could use the HubSpot API to retrieve contact information and use it to trigger personalized email campaigns based on a contact's behavior on the company's website.
This article shows you how to use the Python programming to manage contacts on HubSpot. It offers detailed steps to efficiently access, retrieve, and generate contacts on the HubSpot platform using Python.
The code used in this tutorial can be found in this GitHub repository.
Setting Up Your HubSpot Account
Before getting started on the tutorial, you need to get your HubSpot account set up.
If you don't have a HubSpot account, sign up for a free or demo account on the HubSpot website.
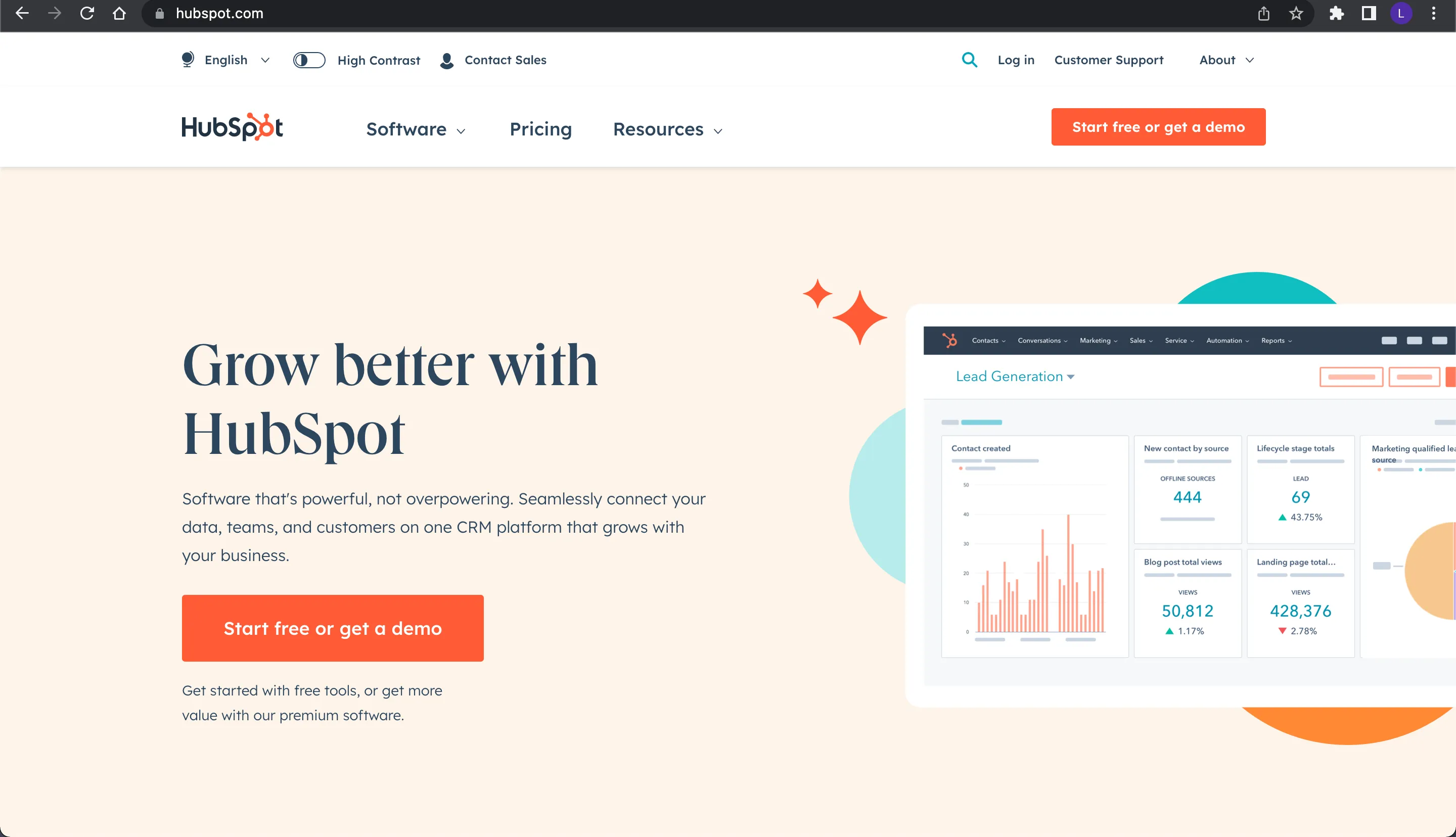
Follow the instructions to sign up. Once your account is created, you'll be logged in to HubSpot. If you already have an account, simply log in.

Create Contacts
Next, you need to create several contacts to be used later in this tutorial. Use this exercise to also familiarize yourself with the HubSpot platform.
To create a new contact, navigate to Contacts from the top navigation bar, then click on Create contact in the top right corner of the page. Fill out the form with the necessary information, including first name, last name, and email. Click on the Create button to submit the contact.

To delete a contact, click on the contact in the list of contacts in the HubSpot dashboard. Click on Actions and then Delete to remove the contact permanently.
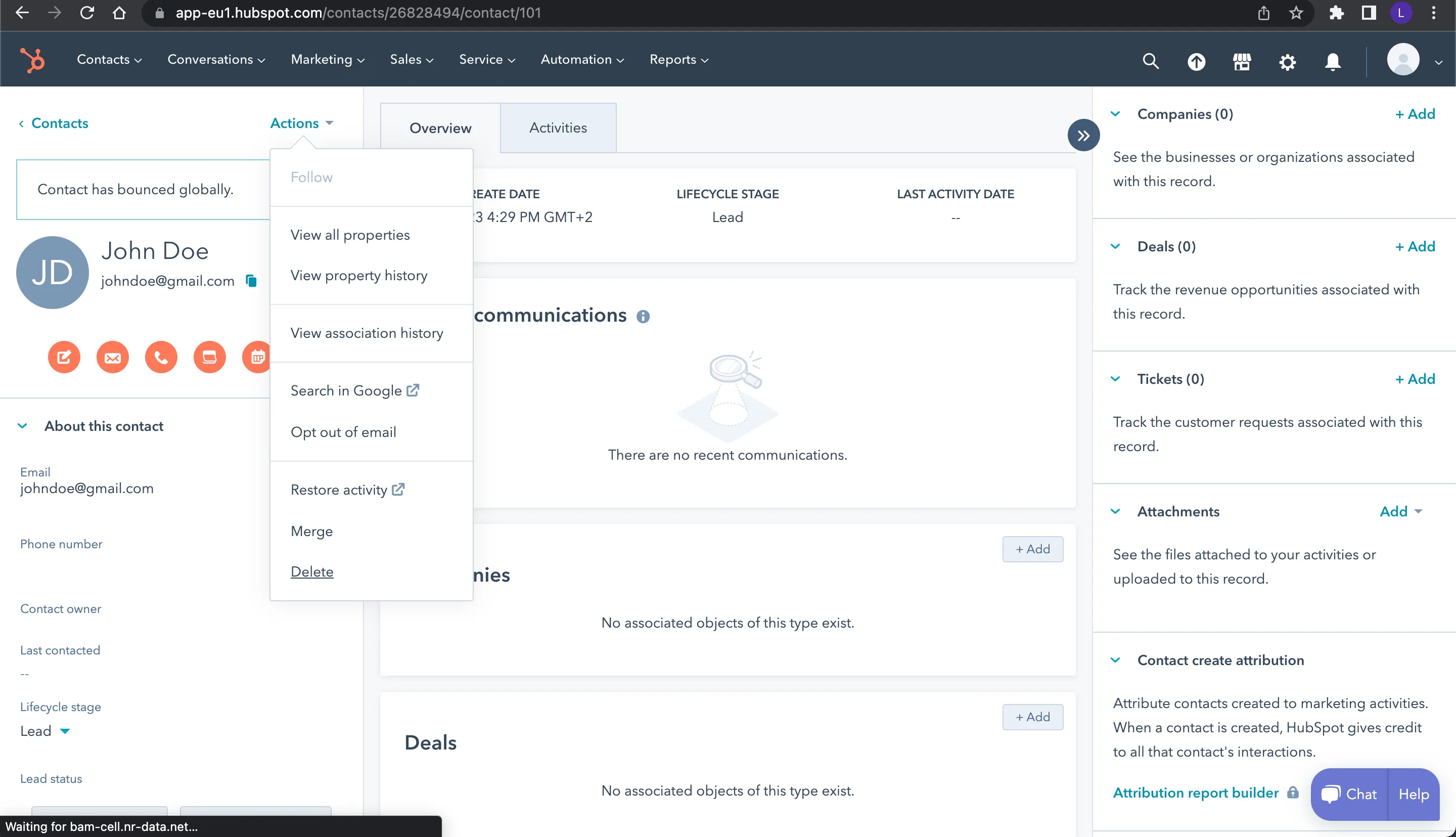
Create four to five contacts with whatever details you wish to use later in this tutorial.
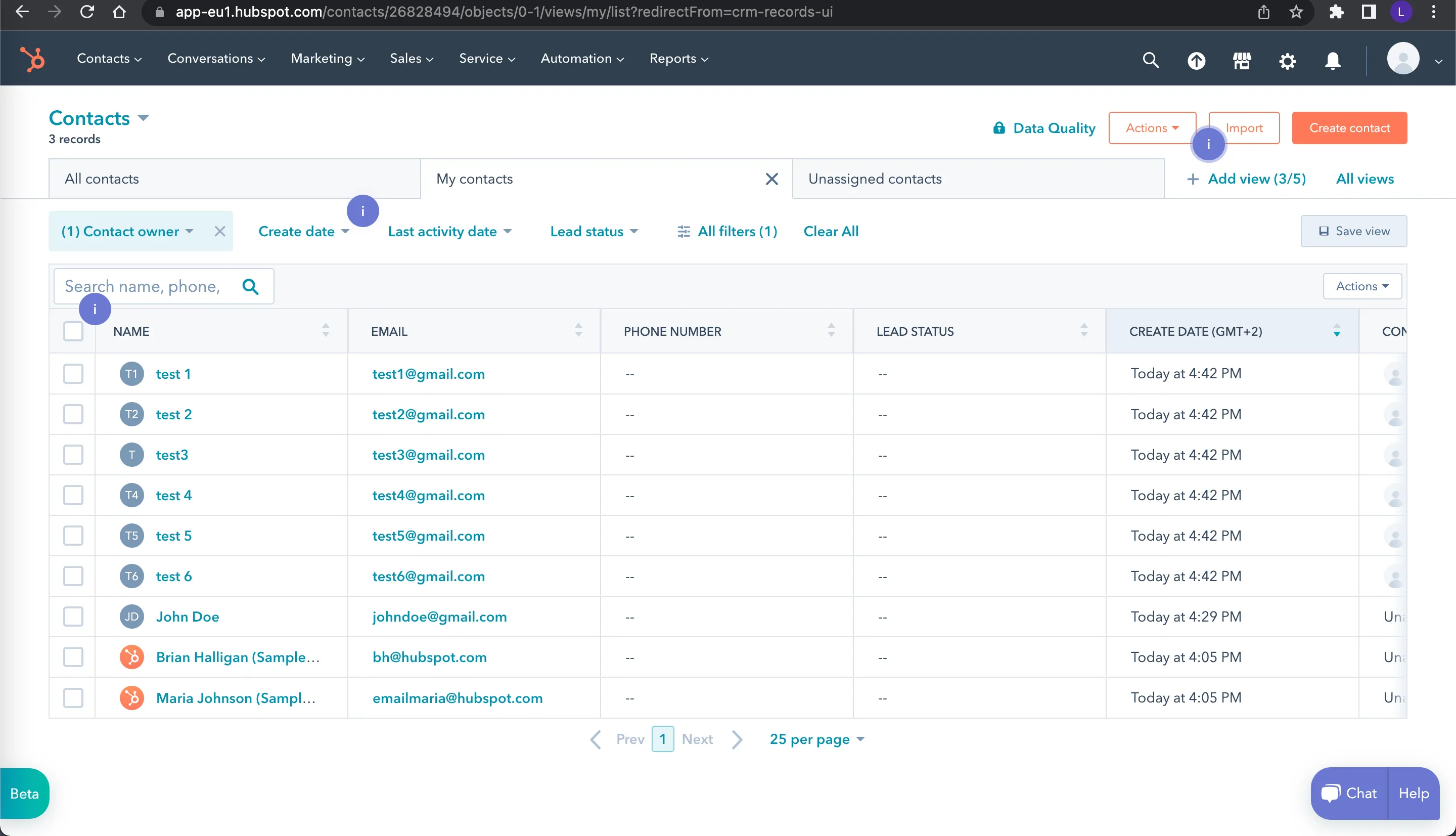
Create a HubSpot App and Generate an Application Token
Lastly, you need to create a HubSpot app to get a token for authenticating requests made to your HubSpot account, which you'll use later in the tutorial.
Click on Settings in the top navigation bar, then navigate to the Private Apps section under Integrations. Click on Create a private app and fill in the information required.
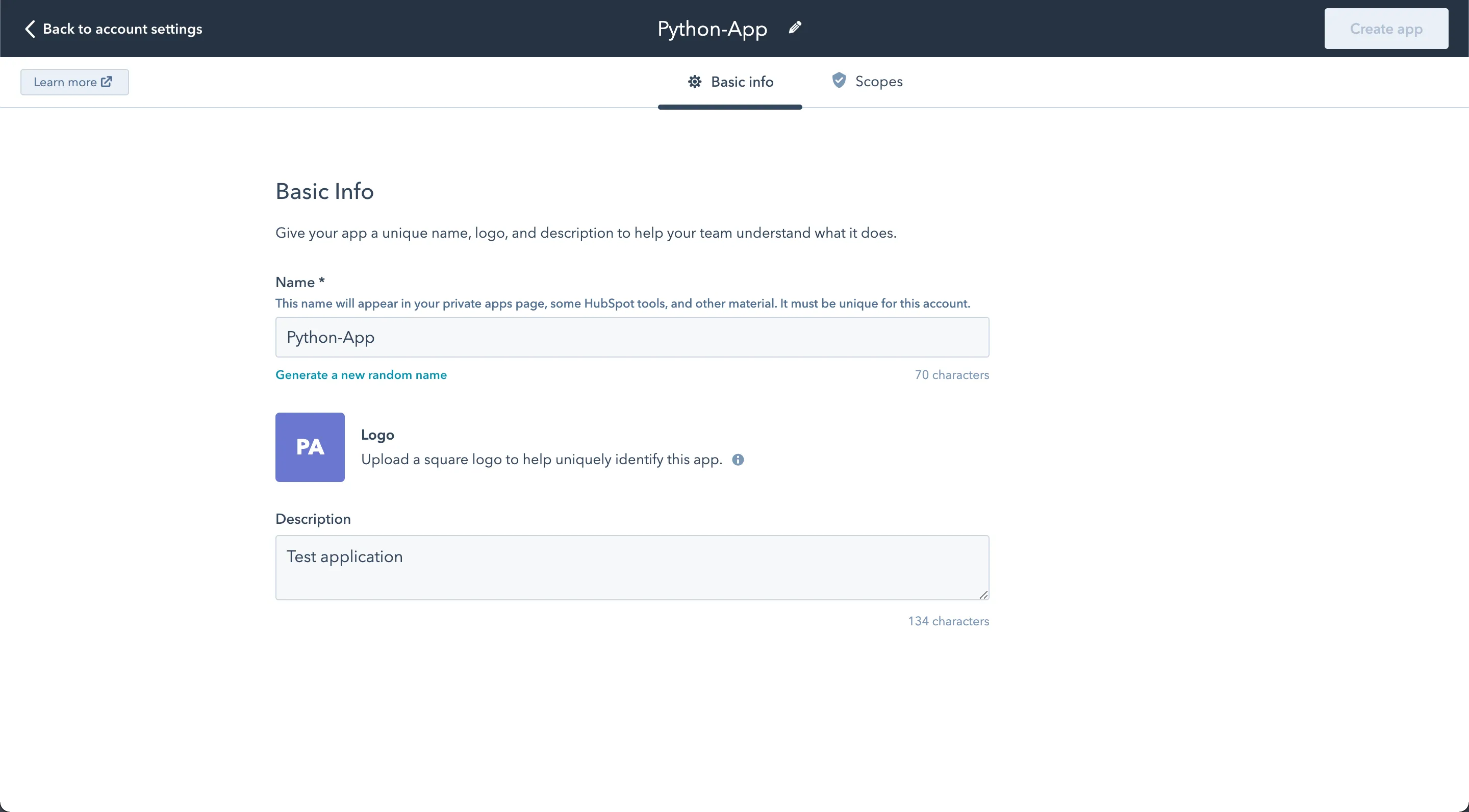
Next, toggle to Scopes, search for contacts, then check all the boxes for Read and Write related to contacts. This will give your application permission to have access to your contacts.

Lastly, click on Create app in the top right corner.
You should get a prompt that states Your private app was created. This prompt contains your application token. Click on Copy and save the token somewhere safe because you'll use it later.
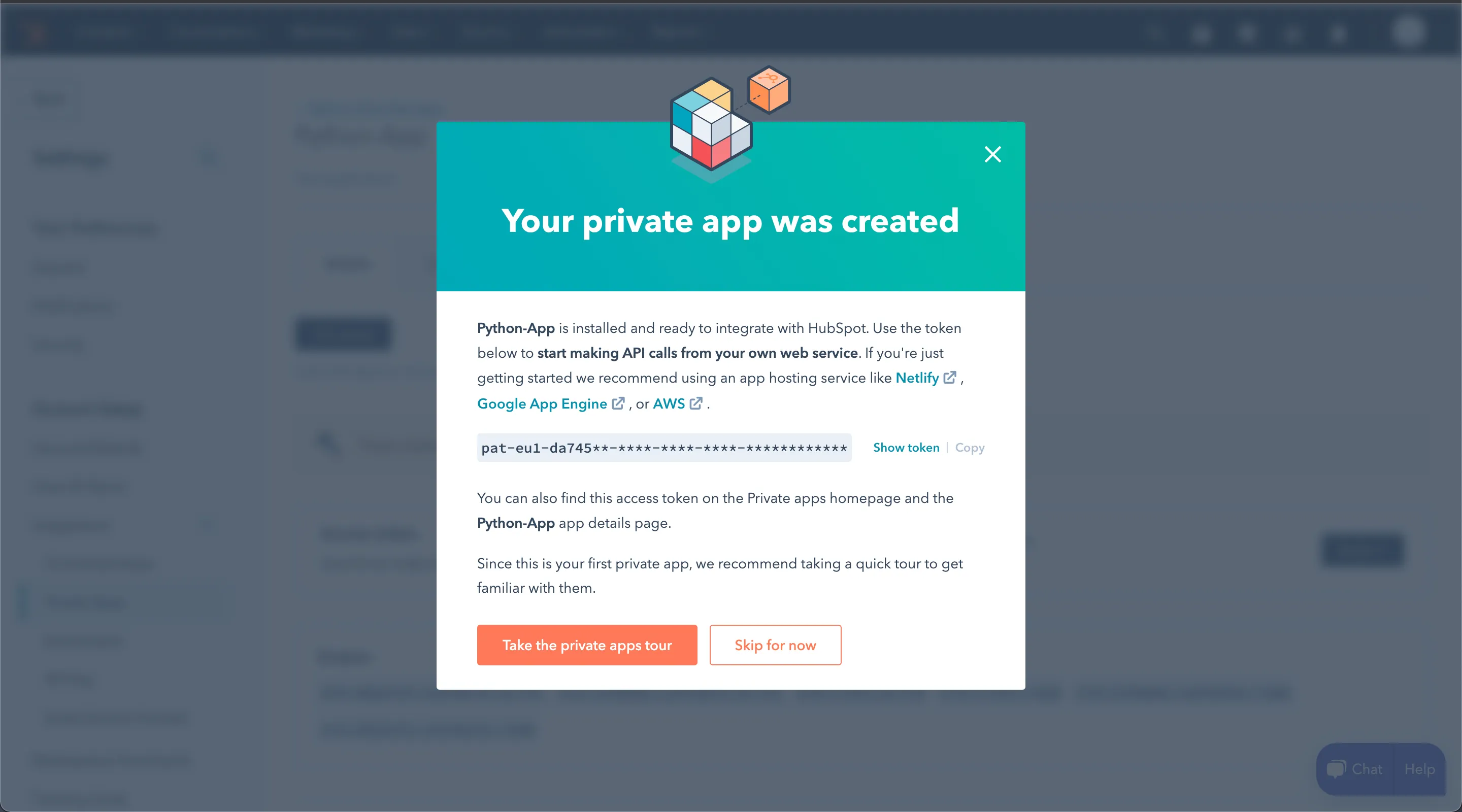
Using Python with HubSpot
With all the setup out of the way, you can now start the tutorial.
Prerequisites
To follow along with this tutorial, you need the following installed on your machine:
- Python 3.11.1
- The Python package installer pip
Once you have these installed, go to your terminal or shell and install the requests library using the following command:
Note: You might have already installed this library with Python. To avoid any issues, it's best to make sure it's installed before proceeding to the next step.
Getting HubSpot Contacts Using the requests.get Method
Create a file named <code class="blog_inline-code">get_hubspot_contacts.py</code> and add the following code to it:
Replace <code class="blog_inline-code">Your_Token</code> with the token you got from the previous step.
In the code above, you start by importing the needed library, requests, to make the HTTP call. You then specify the endpoint URL and the headers that are related to your HubSpot account. Next, you call the HubSpot endpoint using the <code class="blog_inline-code">request.get()</code> method, and finally, if the result is successful, you display the contacts by using a <code class="blog_inline-code">for loop</code> and the <code class="blog_inline-code">print()</code> function.
Run the code from the terminal or command line using the following command:
You should see the contacts you created on HubSpot as the output.

Creating HubSpot Contacts Using the requests.post Method
Create a file named <code class="blog_inline-code">create_hubspot_contact.py</code> and add the following code to it:
Replace <code class="blog_inline-code">Your_Token</code> with the token you got earlier.
In the code above, you again start by importing the needed library, requests, to make the HTTP call, and then you specify the endpoint URL and the headers that are related to your HubSpot account. Next, you define the contact information that you want to create, after which you call the HubSpot endpoint using the <code class="blog_inline-code">request.post()</code> method. If the result is successful, you should see the contact created on your HubSpot contacts.
Run the code from the terminal or command line using the following command:
You should see "Contact Created Successfully" as the output.

If you check the HubSpot portal, you should see your newly created contact.
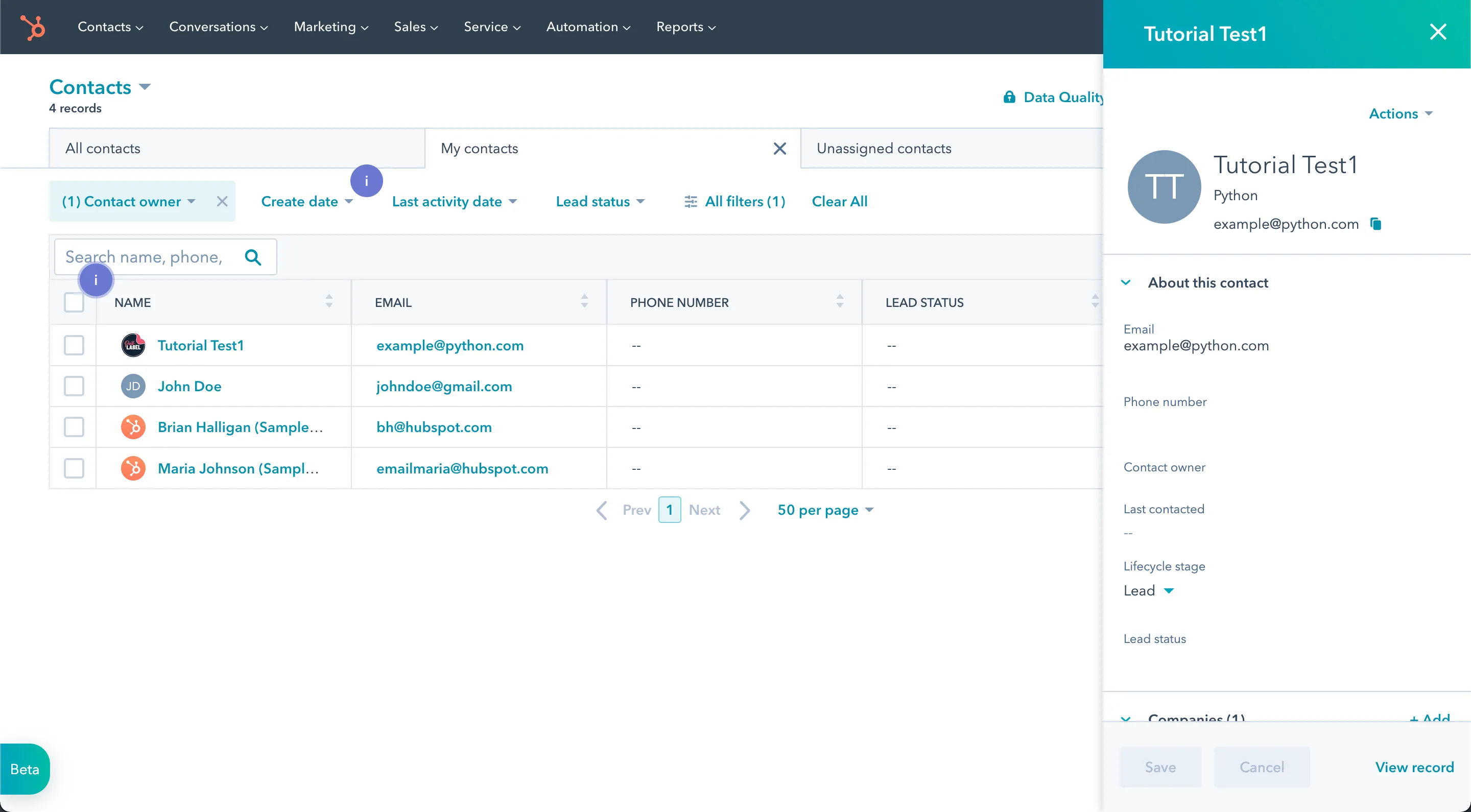
Creating a Webhook Using Python to Fetch HubSpot Contacts
If you want to automate the process of retrieving new or updated contact information and integrating it with other systems or applications, using a webhook with Python to fetch HubSpot contacts can be useful.
In this section, you'll use Flask to create an endpoint that will serve as an API, or webhook, to call to get contacts. Flask is a lightweight Python framework used to create APIs.
Install the Flask library with the following command:
Next, create a file named <code class="blog_inline-code">app.py</code> and add the following code to it:
Remember to replace <code class="blog_inline-code">Your_Token</code> with the token you got earlier.
In the code above, you start by importing the <code class="blog_inline-code">requests</code> library to make the HTTP call and the <code class="blog_inline-code">flask</code> library to create and run your endpoint. You then define the route for the HTTP GET request of the endpoint, which is <code class="blog_inline-code">/contacts</code>. The route calls the <code class="blog_inline-code">get_contacts()</code> function, which calls the HubSpot endpoint and returns the contacts. This route defines the endpoint URL and the headers related to your HubSpot account.
Run the code from the terminal or command line using the following command:

Next, open your browser and paste in the following URL: http://localhost:5000/contacts. You should see the contacts displayed in your browser.

Managing Integrations with Merge's Unified API
But what if you are not proficient in programming languages like Python? Or what if HubSpot is just one of several integrations you and your team need to build and maintain?
That's where a tool like Merge can help.
Merge offers a unified API for all suites of integrations by providing a single API endpoint that connects to multiple platforms and services—including HubSpot. The API endpoint acts as a bridge between the different platforms and services, allowing users to interact with all of them using a single API call.
To achieve this, Merge uses a system of plugins that allows it to connect to different platforms and services. These plugins act as a bridge between the Merge API endpoint and the various platforms and services. Each plugin is responsible for handling the specific communication protocols, APIs, and data structures of the platform or service it connects to.
When a user makes an API call to Merge, the call is routed to the appropriate plugin based on the information provided in the call. The plugin then processes the call and returns the appropriate response. This allows users to interact with all the different platforms and services through a single, unified API endpoint.
Merge also allows developers to build custom plugins to connect to new platforms and services, which can be added to the API endpoint. This means that Merge is constantly expanding its integration capabilities and support for new platforms and services.
Conclusion
This article showed you how to use Python to interact with the HubSpot CRM to fetch and create contacts. You learned how to create a webhook to automatically retrieve HubSpot contacts when the endpoint is accessed.
You also learned how the Merge CRM Unified API allows you to connect with hundreds platforms, including HubSpot and Salesforce, through a single call, eliminating the need for multiple integrations. Simplifying the process of integrating with various tools and services allows you to focus on your core product, leading to increased efficiency and improved bottom line.
Check out our Guide to CRM Integrations for examples of product integrations, an overview of CRM data schemas, and other useful information to consider as you work with CRM APIs.
Learn more about Merge by scheduling a demo with one of our integration experts.